Spotlights on Base Python
Overview and the Very Basis
Base Python is the foundational aspect of the Python programming language, encompassing its syntax, semantics, and basic features. Understanding these fundamentals is crucial for any programmer, as they form the building blocks for writing efficient and effective code. This section will cover the essential concepts and the core philosophy behind Python, including its readability and simplicity.
Basic Data Types and Variables
Python supports several built-in data types, which are essential for storing and manipulating data. The primary data types include:
- Integers: Whole numbers, e.g.,
5
,-3
. - Floats: Decimal numbers, e.g.,
3.14
,-0.001
. - Strings: Sequences of characters, e.g.,
"Hello, World!"
. - Booleans: Represents
True
orFalse
values.
Variables in Python are created by assigning a value to a name, which can then be used to store and manipulate data throughout the program.
Control Structures
Control structures determine the flow of a program’s execution. In Python, the primary control structures include:
- Conditional Statements: Use
if
,elif
, andelse
to execute different blocks of code based on certain conditions. - Loops: Use
for
andwhile
loops to iterate over sequences or execute code repeatedly until a condition is met.
Understanding control structures is essential for creating dynamic and responsive programs.
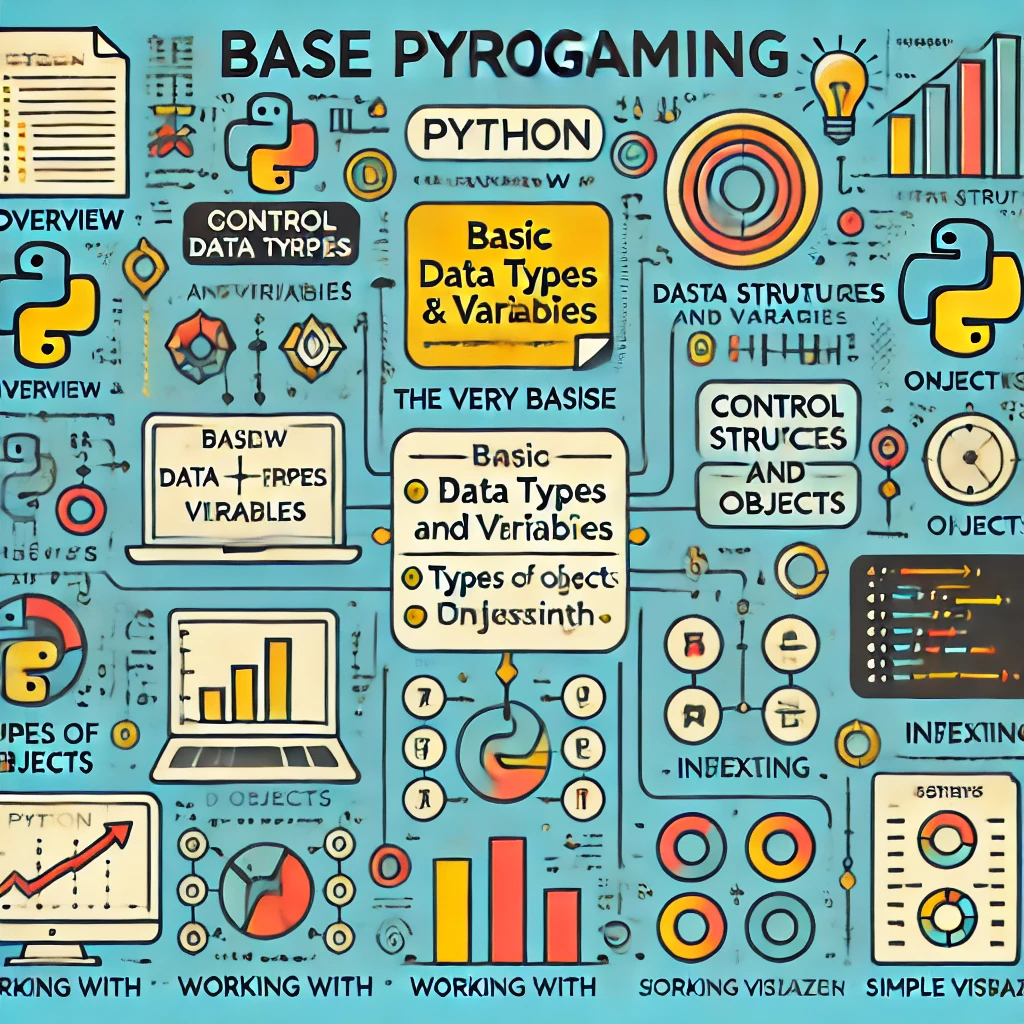
Types of Objects
Python is an object-oriented language, meaning everything in Python is an object. Different types of objects include:
- Lists: Ordered collections of items, e.g.,
[1, 2, 3]
. - Tuples: Immutable ordered collections, e.g.,
(1, 2, 3)
. - Dictionaries: Unordered collections of key-value pairs, e.g.,
{"name": "Alice", "age": 25}
. - Sets: Unordered collections of unique items, e.g.,
{1, 2, 3}
.
Each type of object has its own methods and properties, allowing for versatile data manipulation.
Indexing
Indexing refers to accessing elements in sequences such as lists, strings, and tuples. In Python, indexing starts at 0
, which means:
- The first element can be accessed with
my_list[0]
. - Negative indexing allows access to elements from the end of the sequence, e.g.,
my_list[-1]
refers to the last element.
Mastering indexing is essential for effective data manipulation and retrieval.
Working With
Working with data in Python involves using functions and methods to manipulate and analyze data. This includes:
- Built-in Functions: Functions like
len()
,sum()
, andmax()
to perform operations on data. - Methods: Object-specific functions, e.g.,
list.append()
,str.lower()
, that provide additional functionality.
Understanding how to work with these functions and methods is critical for efficient programming.
Simple Visualization
Data visualization in Python can be accomplished using libraries such as Matplotlib and Seaborn. Basic visualizations include:
- Line Plots: Displaying data points connected by lines.
- Bar Charts: Representing data with rectangular bars.
- Scatter Plots: Showing the relationship between two numerical variables.
Visualizing data helps in understanding patterns, trends, and insights that can inform decision-making.
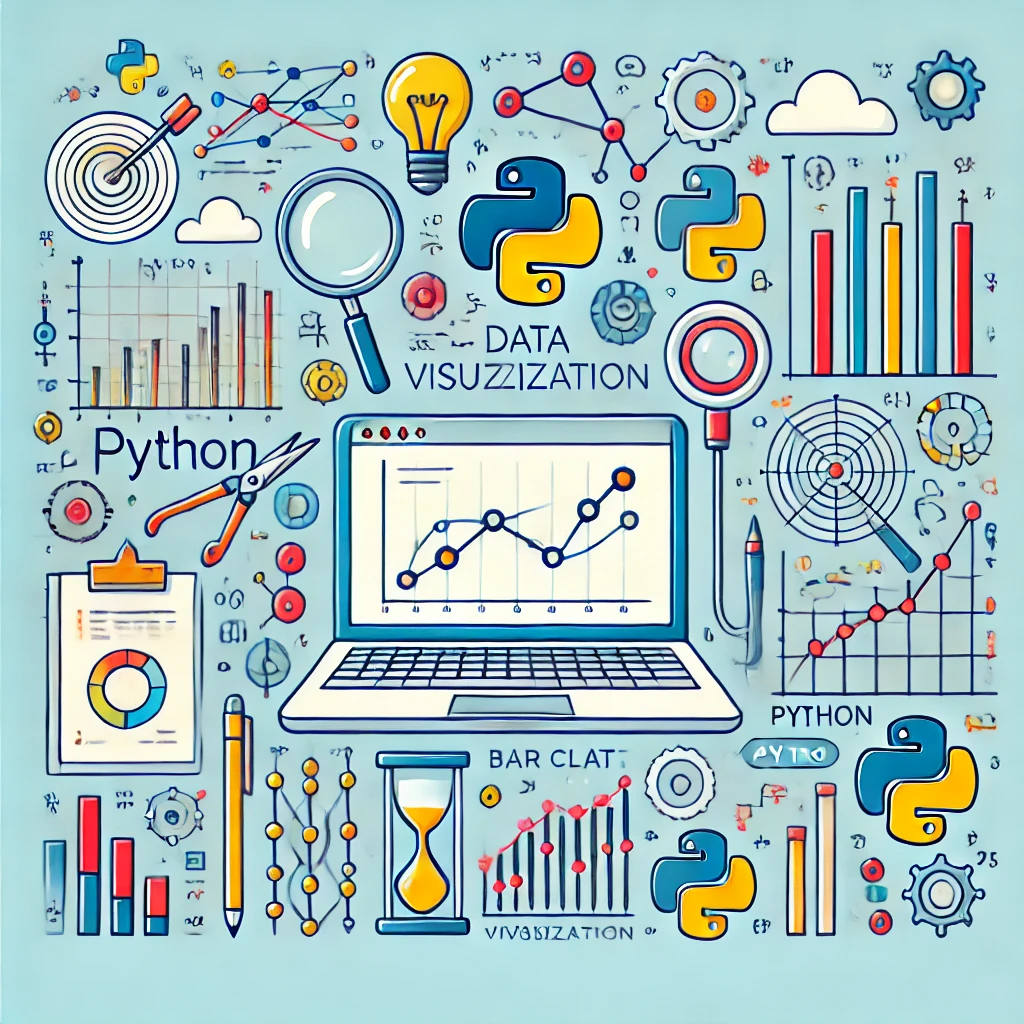