Cheat sheet
On this page you will find a collection of useful pdf files and code snippets.
RStudio IDE Cheat Sheet
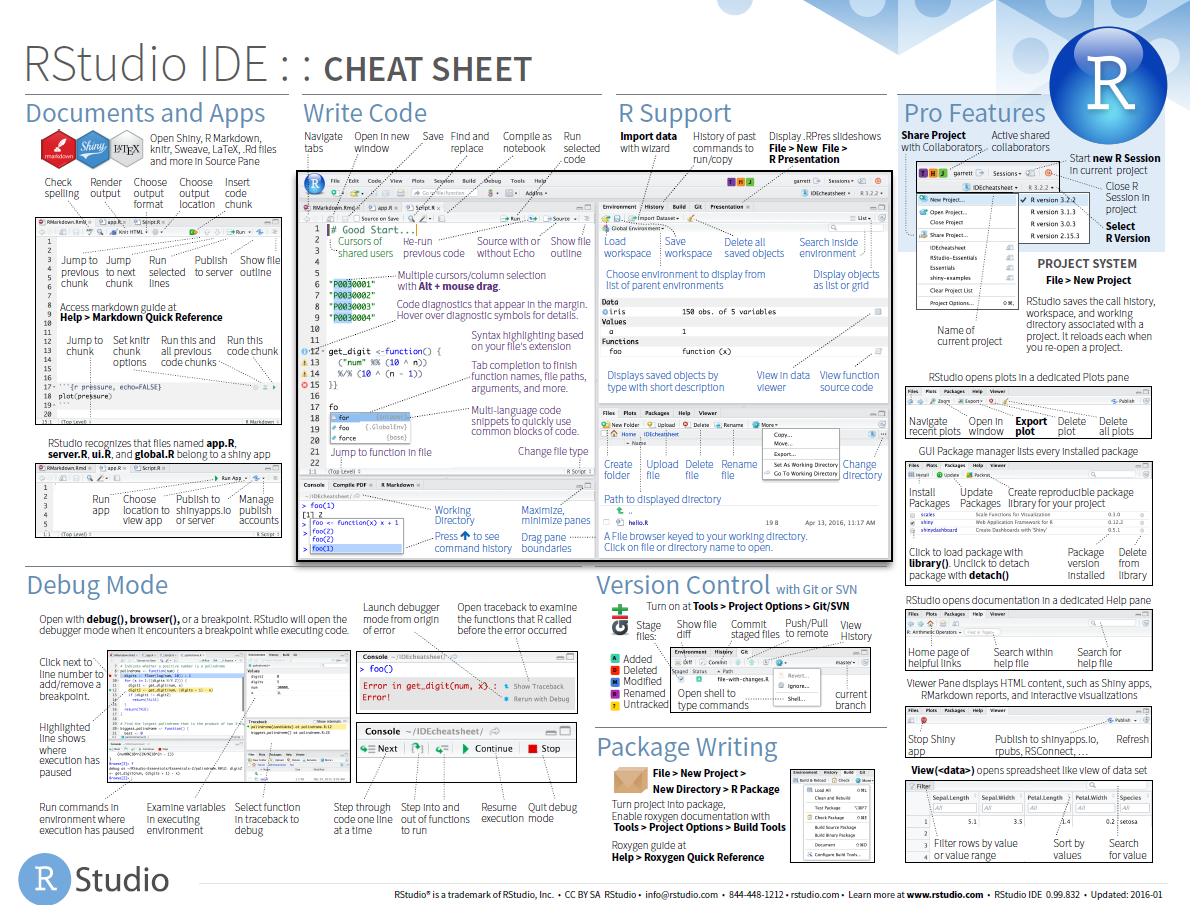
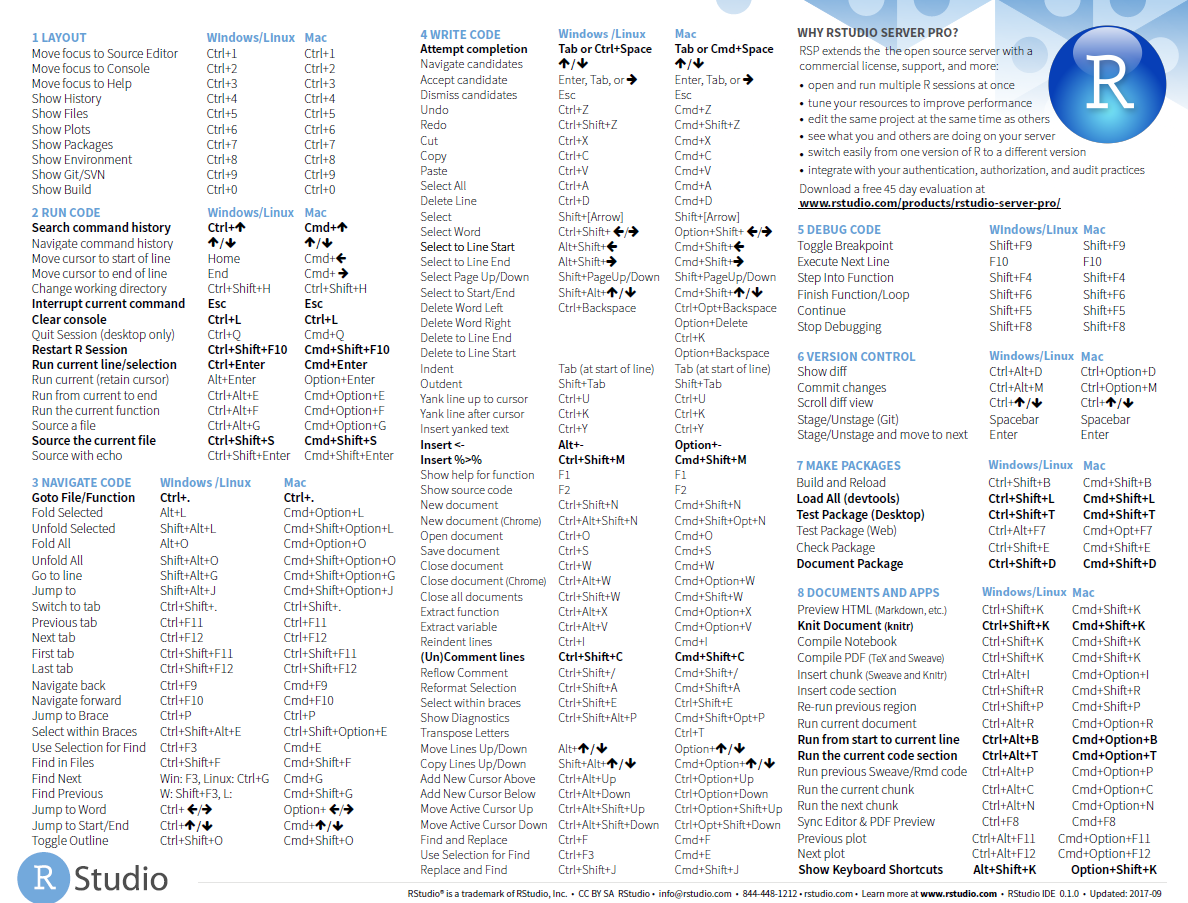
Download RStudio IDE Cheat Sheet
Base R Cheat Sheet
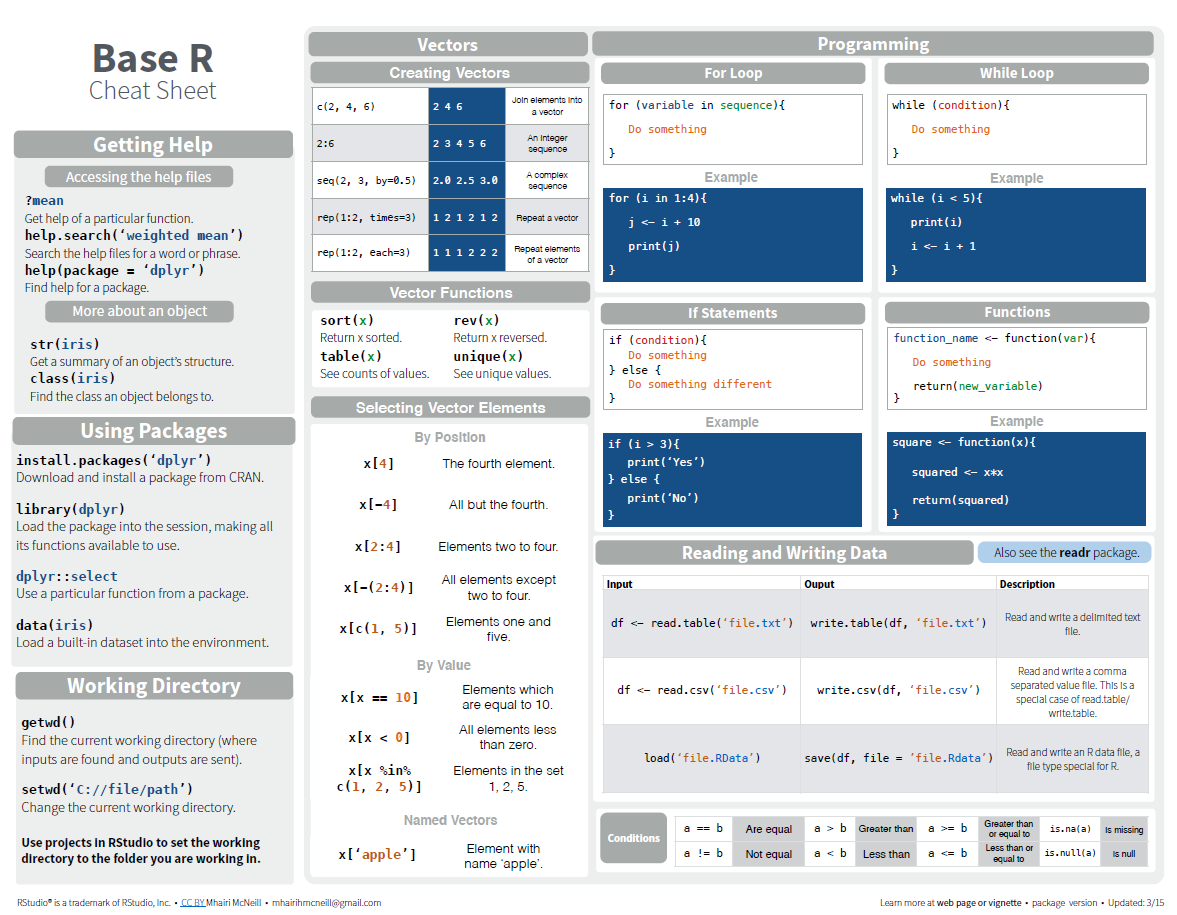
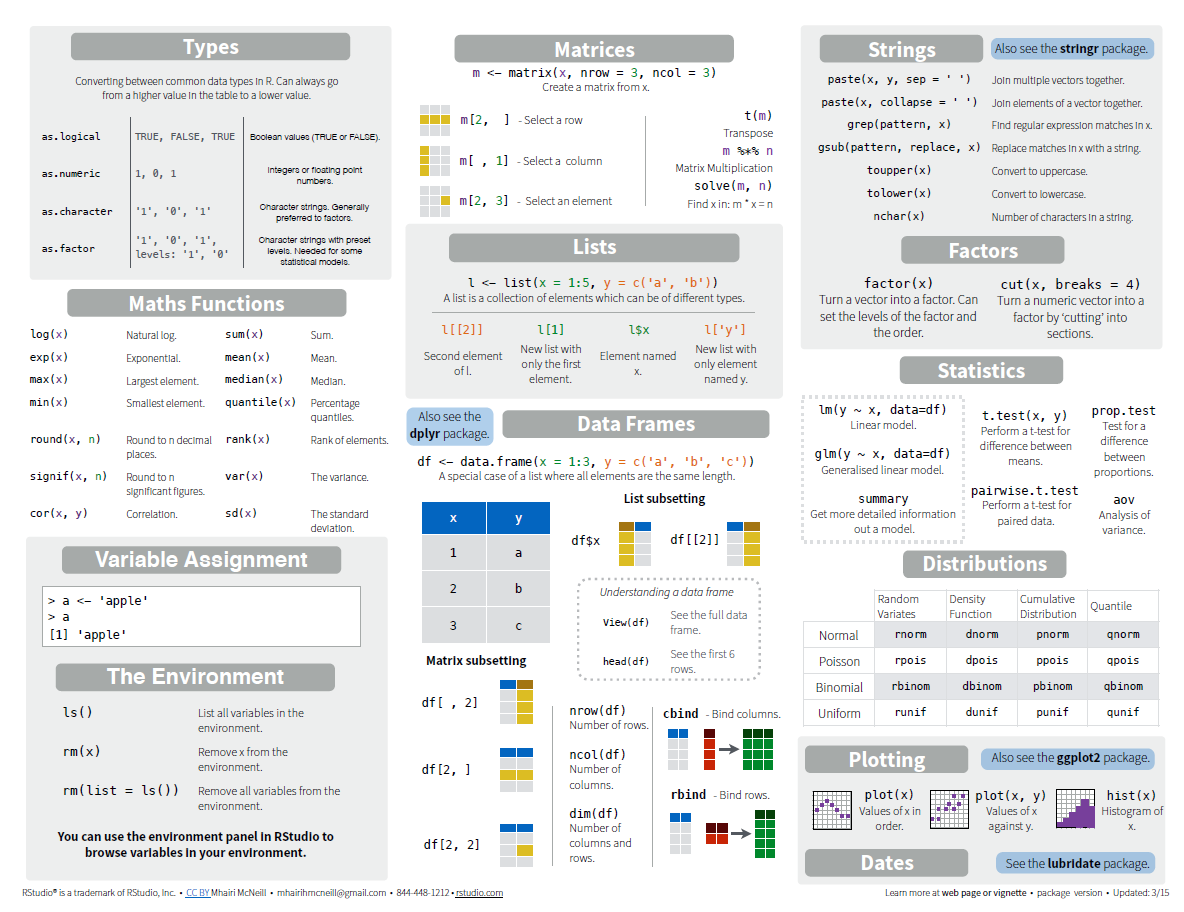
Advanced R Cheat Sheet
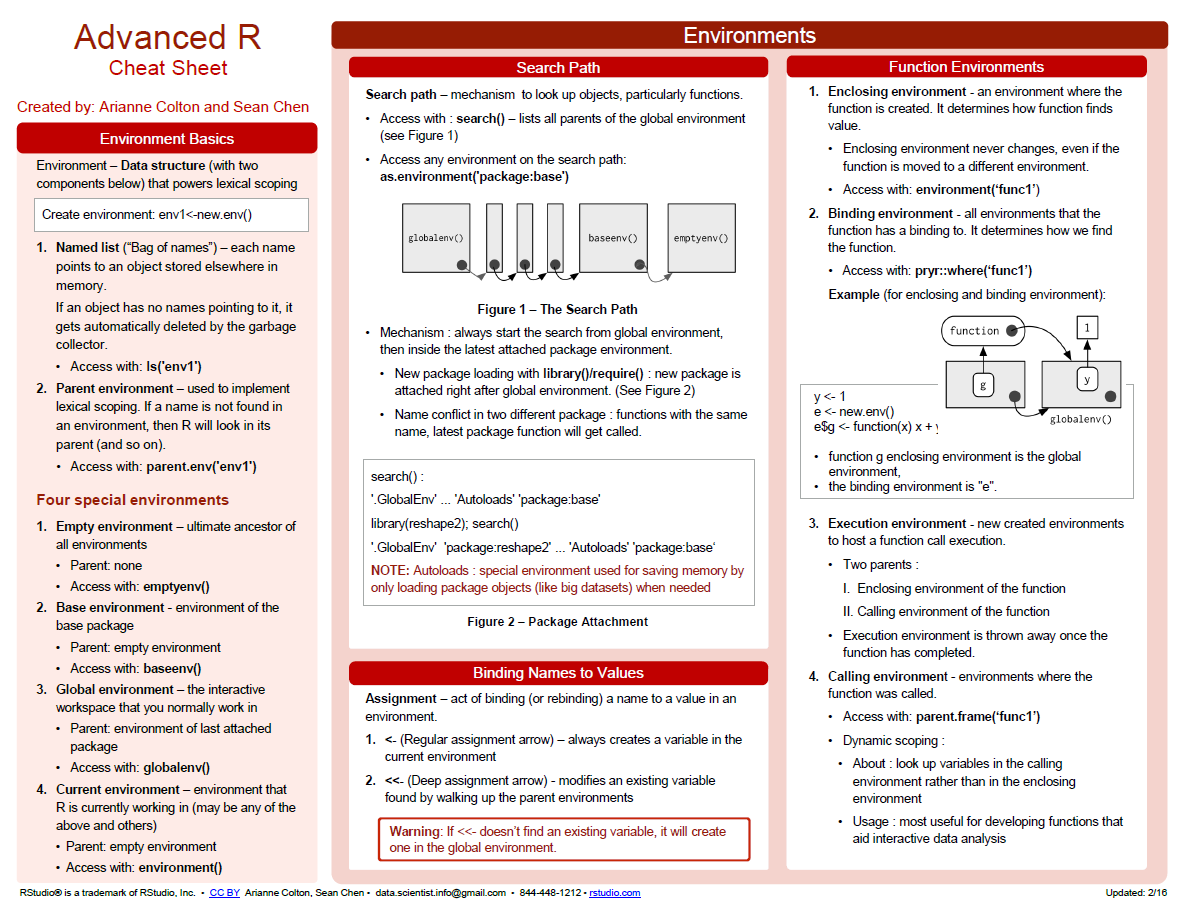
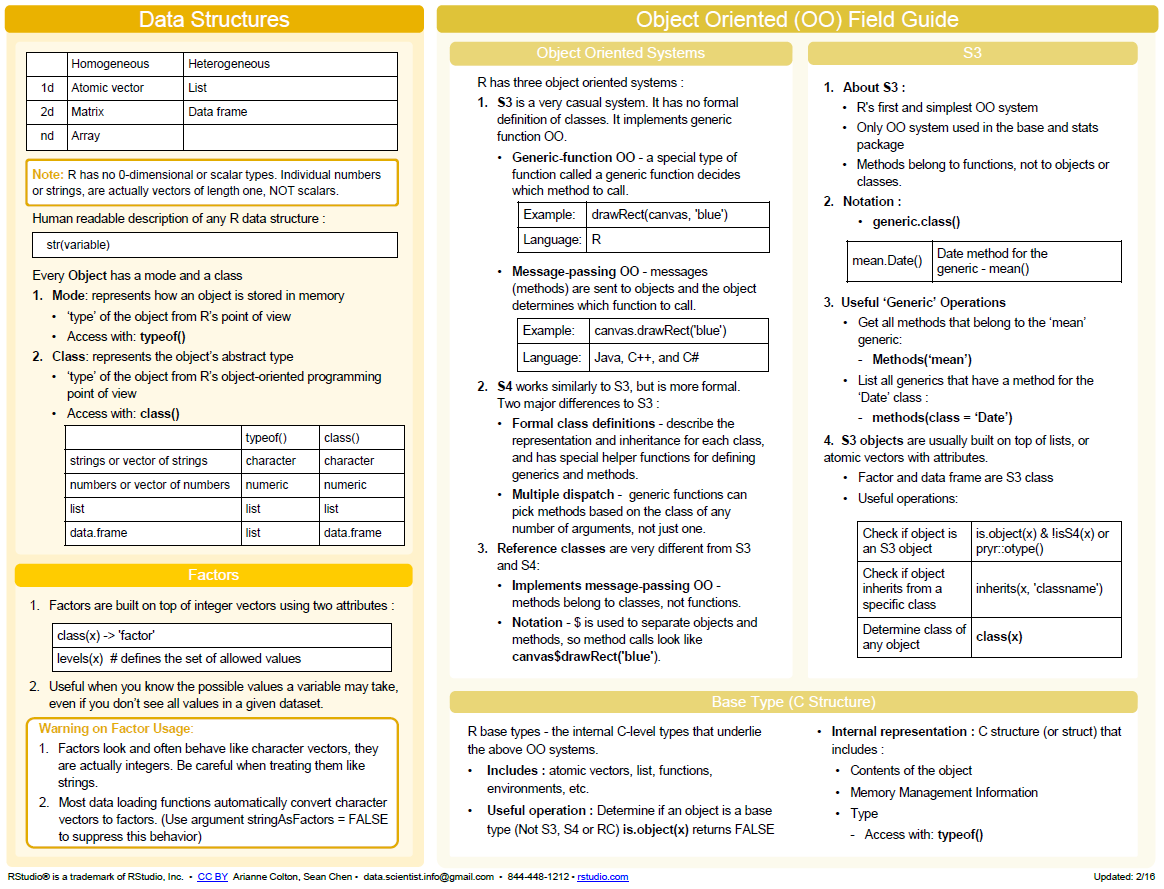
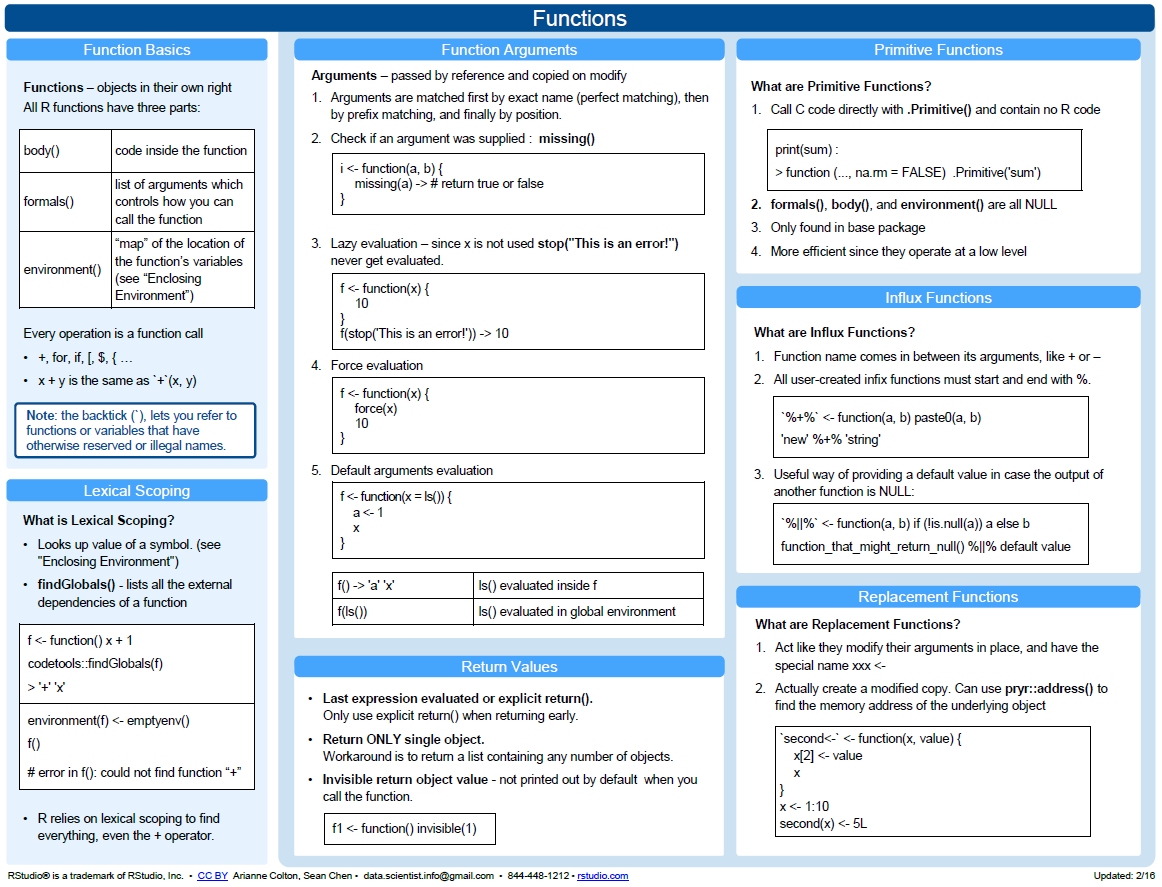
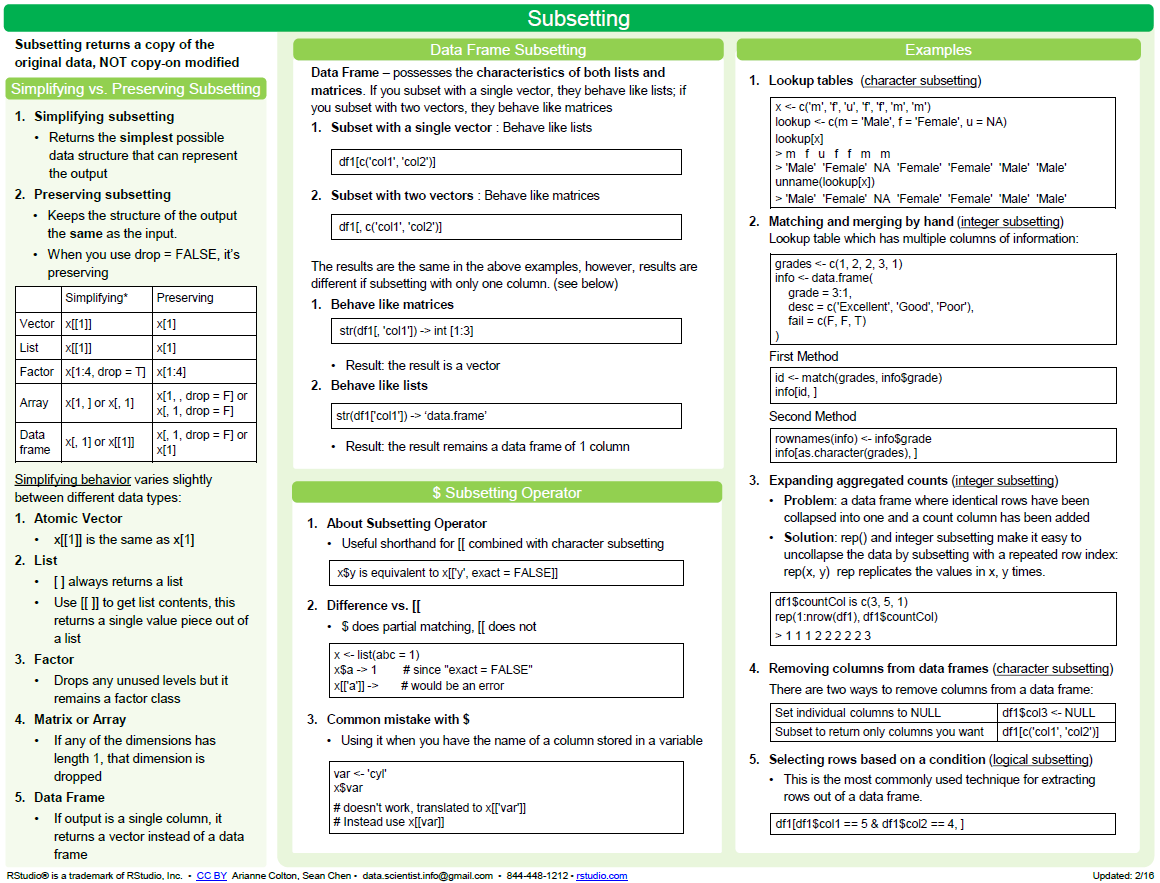
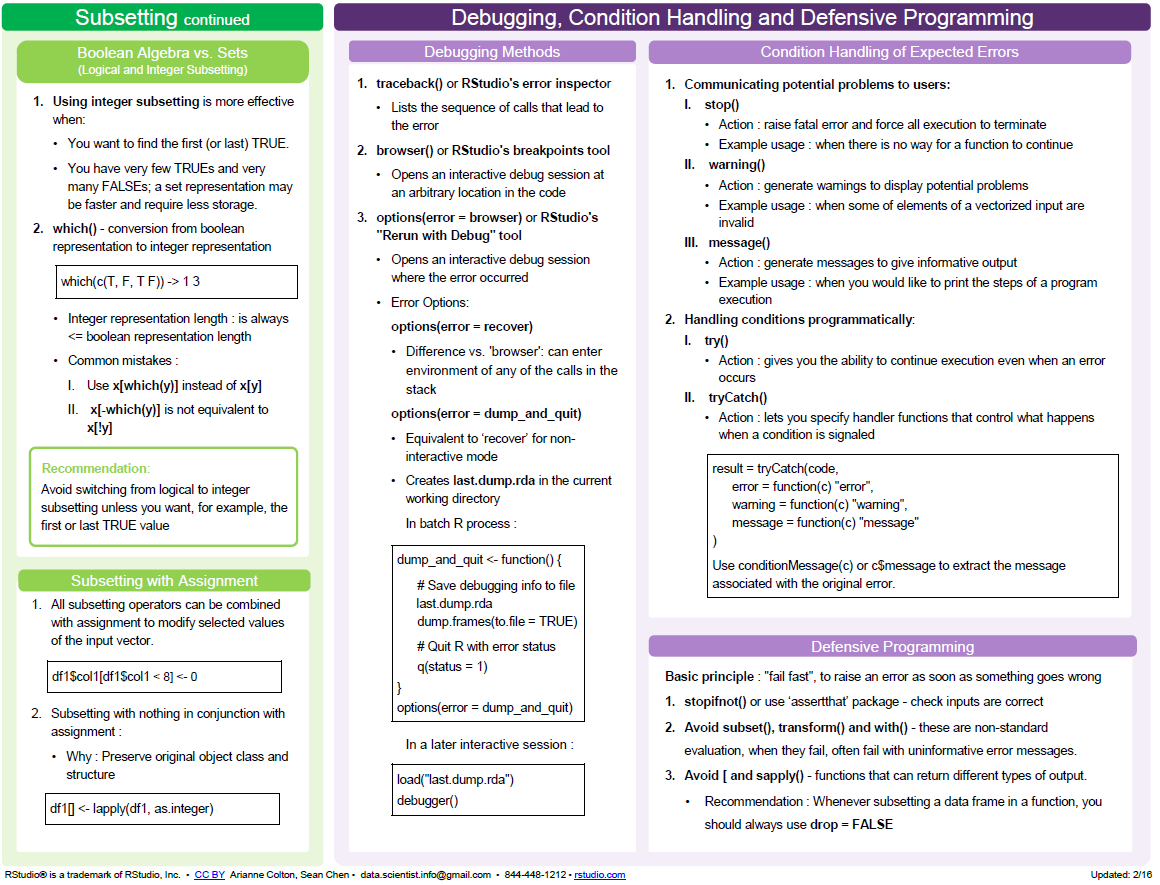
On this page you will find a collection of useful pdf files and code snippets.
Download RStudio IDE Cheat Sheet